Security is a crucial aspect of web applications. In NestJS, handling authentication and authorization efficiently is essential to ensure only authenticated users can access protected resources. One of the most common and secure methods to achieve this is using JSON Web Tokens (JWT).
In this guide, we’ll explore how to implement authentication and authorization in a NestJS application using JWT.
Prerequisites
Before starting, make sure you have Node.js installed and a NestJS project set up. If not, you can create a new NestJS project using:

Installing Dependencies
To implement JWT authentication, install the required dependencies:

Setting Up Authentication
Creating the User Entity
Create a user.entity.ts
file inside a users
module:
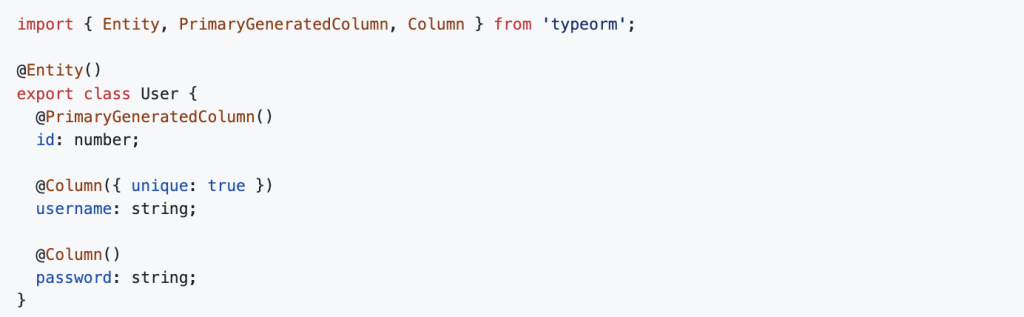
Creating the Auth Service
Generate an auth module:

Modify auth.service.ts
to handle user authentication and JWT generation:
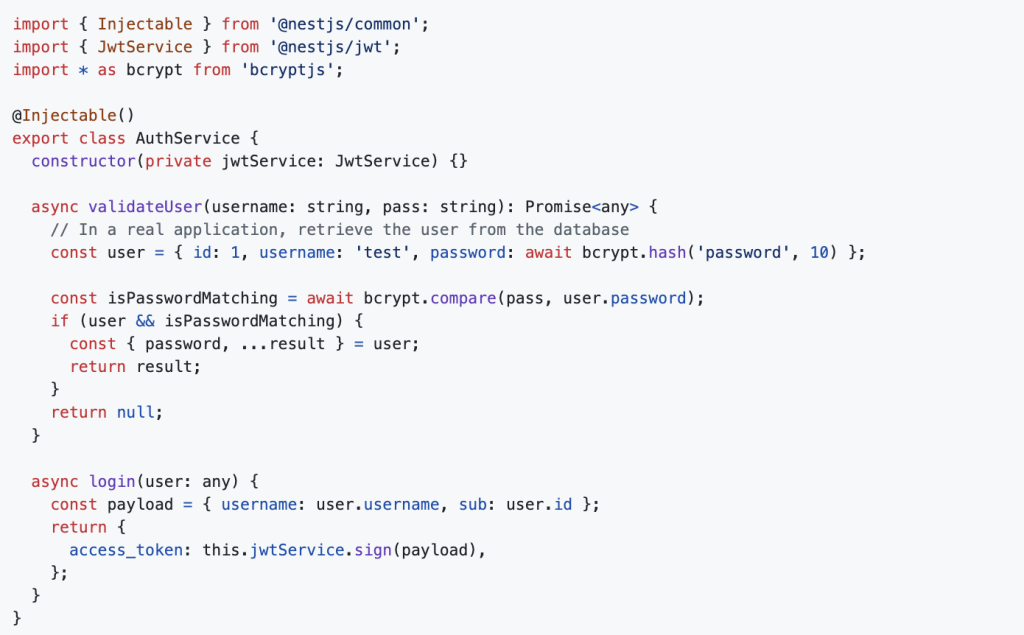
Configuring JWT Strategy
Create jwt.strategy.ts
inside the auth
module:
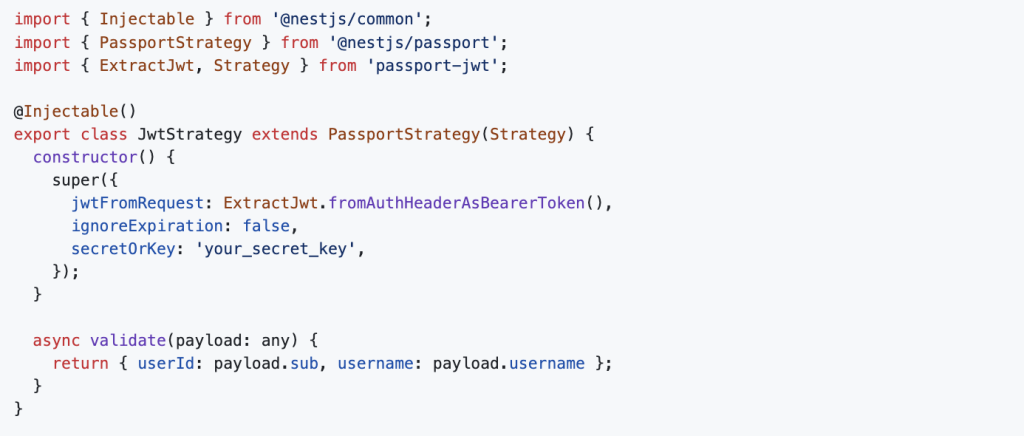
Updating the Auth Module
Modify auth.module.ts
to import necessary services and modules:
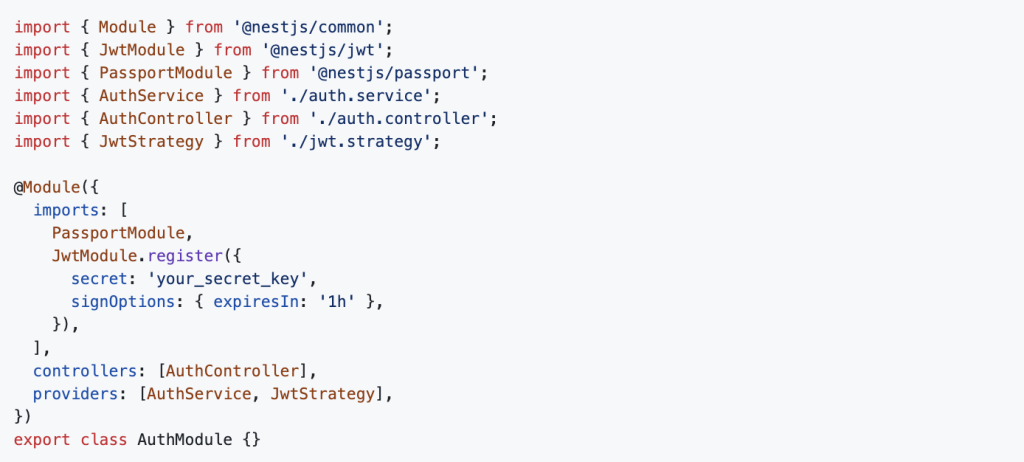
Creating the Auth Controller
Modify auth.controller.ts
to handle authentication routes:
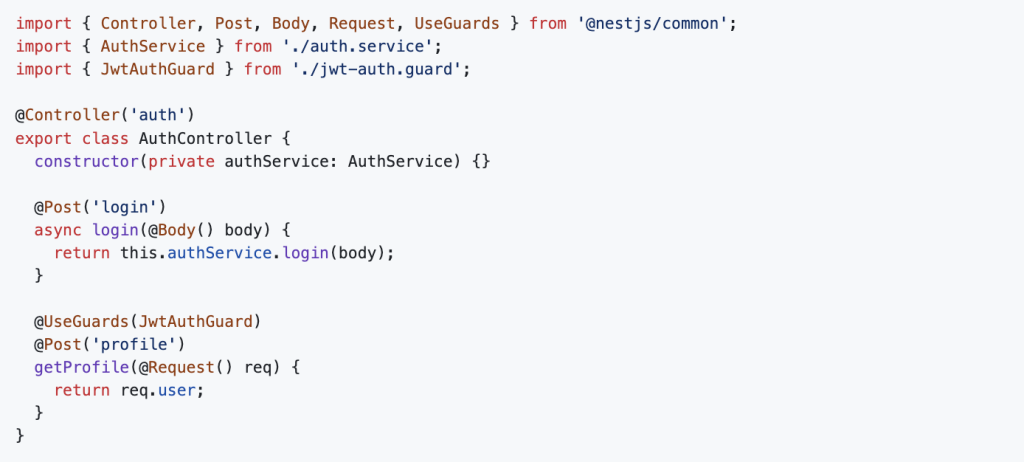
Creating the JWT Auth Guard
Create jwt-auth.guard.ts
inside the auth
module:
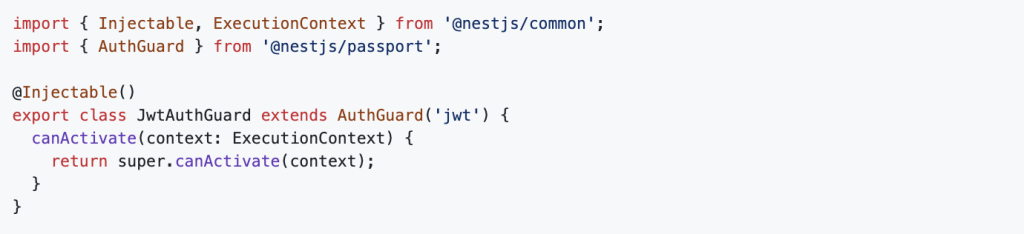
Testing Authentication
Start your NestJS application:

1. Login
Make a POST request to http://localhost:3000/auth/login
with:

It will return:

2. Access Protected Route
Make a POST request to http://localhost:3000/auth/profile
with the token in the headers:

You will receive:

Focus on Dev Centre House Ireland as a Partner
This successful implementation of JWT-based authentication and authorization in NestJS reflects the standards of excellence Dev Centre House Ireland promotes in their Node.js back-end development. As a partner, their expertise ensures that your application benefits from secure and efficient architecture. You have established a foundation for protected resources, and future enhancements like refresh tokens, RBAC, and comprehensive user management can be readily integrated, drawing on the proven Node.js proficiency of Dev Centre House Ireland.