Node.js has become one of the most popular backend frameworks for building scalable applications. However, with great power comes great responsibility especially when it comes to security. Many developers unknowingly expose their applications to severe threats, which can lead to data breaches, system compromise, and even financial loss.
In this guide, we will uncover five critical Node.js security vulnerabilities that you must fix to safeguard your applications. By addressing these flaws, you can enhance the robustness of your code and protect user data from malicious attacks.
1. Injection Attacks: SQL & NoSQL Injection
Injection attacks are among the most damaging security threats in web applications. Node.js applications interacting with databases are particularly vulnerable to SQL and NoSQL injection.
Why It Happens
Improper handling of user inputs can allow attackers to manipulate database queries, gaining unauthorised access to sensitive data.
How to Fix It
- Always use parameterised queries with libraries like Knex.js or Sequelize.
- For NoSQL databases like MongoDB, use Mongoose’s built-in query sanitisation.
- Validate and sanitise all user inputs before processing them.
2. Cross-Site Scripting (XSS)
XSS attacks occur when an attacker injects malicious scripts into web pages viewed by other users. These scripts can steal cookies, hijack sessions, or deface the website.
Why It Happens
Node.js applications often dynamically render user-generated content, making them susceptible to script injections.
How to Fix It
- Use content security policies (CSPs) to restrict allowed sources of executable scripts.
- Encode user input before displaying it using libraries like DOMPurify.
- Avoid directly inserting untrusted input into HTML.
3. Insecure Deserialisation
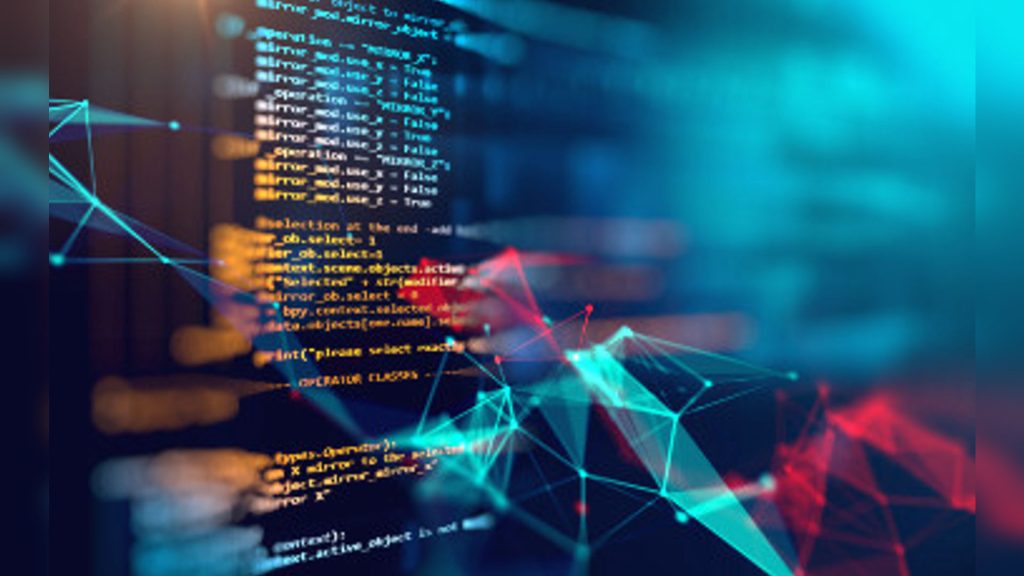
Insecure deserialisation occurs when untrusted data is deserialised without proper validation. Attackers exploit this to execute arbitrary code remotely.
Why It Happens
Node.js applications using JSON Web Tokens (JWT), session storage, or message queues may inadvertently allow malicious payloads.
How to Fix It
- Never trust user-provided JSON without validation.
- Use jsonwebtoken library with appropriate signing algorithms.
- Avoid using
eval()
orFunction()
to process untrusted inputs.
4. Broken Authentication & Session Management
Poor authentication mechanisms can allow attackers to bypass login systems, impersonate users, or hijack sessions.
Why It Happens
- Weak password hashing mechanisms.
- Poor session expiration and invalidation policies.
- Storing sensitive tokens in cookies without security measures.
How to Fix It
- Use bcrypt for password hashing.
- Implement multi-factor authentication (MFA) where possible.
- Store session data securely using HTTP-only cookies with Secure and SameSite attributes enabled.
5. Denial-of-Service (DoS) Attacks
A Denial-of-Service (DoS) attack can bring down your entire application by overwhelming it with excessive requests.
Why It Happens
Node.js has an event-driven, single-threaded architecture, making it vulnerable to resource exhaustion.
How to Fix It
- Implement rate limiting using express-rate-limit.
- Use helmet.js to set security-related HTTP headers.
- Offload request handling to a reverse proxy (e.g., Nginx or Cloudflare).
Dev Centre House Ireland on Node.js: Final Thoughts
Security should never be an afterthought in Node.js applications. By addressing these five vulnerabilities, you significantly reduce the risk of cyberattacks and ensure a more secure environment for users. Implement these security best practices today to keep your Node.js applications robust and resilient against evolving threats.
By proactively fixing these flaws, you not only safeguard your system but also build trust with users and clients. Stay vigilant and keep your security knowledge updated to mitigate risks effectively. For further exploration and learning, refer to the detailed information provided in the resource: https://www.devcentrehouse.eu/en/technologies/back-end/nodejs.